mirror of
https://github.com/ueberdosis/tiptap.git
synced 2024-12-20 23:08:07 +08:00
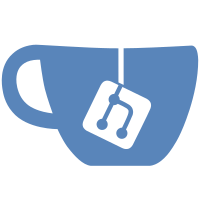
* chore:(core): migrate to tsup * chore: migrate blockquote and bold to tsup * chore: migrated bubble-menu and bullet-list to tsup * chore: migrated more packages to tsup * chore: migrate code and character extensions to tsup * chore: update package.json to simplify build for all packages * chore: move all packages to tsup as a build process * chore: change ci build task * feat(pm): add prosemirror meta package * rfix: resolve issues with build paths & export mappings * docs: update documentation to include notes for @tiptap/pm * chore(pm): update tsconfig * chore(packages): update packages * fix(pm): add package export infos & fix dependencies * chore(general): start moving to pm package as deps * chore: move to tiptap pm package internally * fix(demos): fix demos working with new pm package * fix(tables): fix tables package * fix(tables): fix tables package * chore(demos): pinned typescript version * chore: remove unnecessary tsconfig * chore: fix netlify build * fix(demos): fix package resolving for pm packages * fix(tests): fix package resolving for pm packages * fix(tests): fix package resolving for pm packages * chore(tests): fix tests not running correctly after pm package * chore(pm): add files to files array * chore: update build workflow * chore(tests): increase timeout time back to 12s * chore(docs): update docs * chore(docs): update installation guides & pm information to docs * chore(docs): add link to prosemirror docs * fix(vue-3): add missing build step * chore(docs): comment out cdn link * chore(docs): remove semicolons from docs * chore(docs): remove unnecessary installation note * chore(docs): remove unnecessary installation note
77 lines
2.1 KiB
TypeScript
77 lines
2.1 KiB
TypeScript
import { escapeForRegEx, Range } from '@tiptap/core'
|
|
import { ResolvedPos } from '@tiptap/pm/model'
|
|
|
|
export interface Trigger {
|
|
char: string
|
|
allowSpaces: boolean
|
|
allowedPrefixes: string[] | null
|
|
startOfLine: boolean
|
|
$position: ResolvedPos
|
|
}
|
|
|
|
export type SuggestionMatch = {
|
|
range: Range
|
|
query: string
|
|
text: string
|
|
} | null
|
|
|
|
export function findSuggestionMatch(config: Trigger): SuggestionMatch {
|
|
const {
|
|
char, allowSpaces, allowedPrefixes, startOfLine, $position,
|
|
} = config
|
|
|
|
const escapedChar = escapeForRegEx(char)
|
|
const suffix = new RegExp(`\\s${escapedChar}$`)
|
|
const prefix = startOfLine ? '^' : ''
|
|
const regexp = allowSpaces
|
|
? new RegExp(`${prefix}${escapedChar}.*?(?=\\s${escapedChar}|$)`, 'gm')
|
|
: new RegExp(`${prefix}(?:^)?${escapedChar}[^\\s${escapedChar}]*`, 'gm')
|
|
|
|
const text = $position.nodeBefore?.isText && $position.nodeBefore.text
|
|
|
|
if (!text) {
|
|
return null
|
|
}
|
|
|
|
const textFrom = $position.pos - text.length
|
|
const match = Array.from(text.matchAll(regexp)).pop()
|
|
|
|
if (!match || match.input === undefined || match.index === undefined) {
|
|
return null
|
|
}
|
|
|
|
// JavaScript doesn't have lookbehinds. This hacks a check that first character
|
|
// is a space or the start of the line
|
|
const matchPrefix = match.input.slice(Math.max(0, match.index - 1), match.index)
|
|
const matchPrefixIsAllowed = new RegExp(`^[${allowedPrefixes?.join('')}\0]?$`).test(matchPrefix)
|
|
|
|
if (allowedPrefixes !== null && !matchPrefixIsAllowed) {
|
|
return null
|
|
}
|
|
|
|
// The absolute position of the match in the document
|
|
const from = textFrom + match.index
|
|
let to = from + match[0].length
|
|
|
|
// Edge case handling; if spaces are allowed and we're directly in between
|
|
// two triggers
|
|
if (allowSpaces && suffix.test(text.slice(to - 1, to + 1))) {
|
|
match[0] += ' '
|
|
to += 1
|
|
}
|
|
|
|
// If the $position is located within the matched substring, return that range
|
|
if (from < $position.pos && to >= $position.pos) {
|
|
return {
|
|
range: {
|
|
from,
|
|
to,
|
|
},
|
|
query: match[0].slice(char.length),
|
|
text: match[0],
|
|
}
|
|
}
|
|
|
|
return null
|
|
}
|