mirror of
https://github.com/ueberdosis/tiptap.git
synced 2024-12-04 11:49:02 +08:00
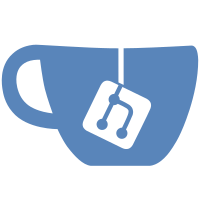
* chore: add precommit hook for eslint fixes, fix linting issues * chore: add eslint import sort plugin
57 lines
1.3 KiB
TypeScript
57 lines
1.3 KiB
TypeScript
/// <reference types="cypress" />
|
|
|
|
import Document from '@tiptap/extension-document'
|
|
import Paragraph from '@tiptap/extension-paragraph'
|
|
import Text from '@tiptap/extension-text'
|
|
import TextAlign from '@tiptap/extension-text-align'
|
|
import { generateJSON } from '@tiptap/html'
|
|
|
|
describe('generateJSON', () => {
|
|
it('generate JSON from HTML without an editor instance', () => {
|
|
const html = '<p>Example Text</p>'
|
|
|
|
const json = generateJSON(html, [
|
|
Document,
|
|
Paragraph,
|
|
Text,
|
|
])
|
|
|
|
expect(JSON.stringify(json)).to.eq(JSON.stringify({
|
|
type: 'doc',
|
|
content: [{
|
|
type: 'paragraph',
|
|
content: [{
|
|
type: 'text',
|
|
text: 'Example Text',
|
|
}],
|
|
}],
|
|
}))
|
|
})
|
|
|
|
// issue: https://github.com/ueberdosis/tiptap/issues/1601
|
|
it('generate JSON with style attributes', () => {
|
|
const html = '<p style="text-align: center;">Example Text</p>'
|
|
|
|
const json = generateJSON(html, [
|
|
Document,
|
|
Paragraph,
|
|
Text,
|
|
TextAlign.configure({ types: ['paragraph'] }),
|
|
])
|
|
|
|
expect(JSON.stringify(json)).to.eq(JSON.stringify({
|
|
type: 'doc',
|
|
content: [{
|
|
type: 'paragraph',
|
|
attrs: {
|
|
textAlign: 'center',
|
|
},
|
|
content: [{
|
|
type: 'text',
|
|
text: 'Example Text',
|
|
}],
|
|
}],
|
|
}))
|
|
})
|
|
})
|