mirror of
https://github.com/microsoft/vcpkg.git
synced 2024-11-27 08:19:10 +08:00
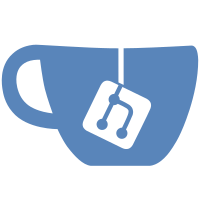
* Fix static qt5 builds and ignore system qmake closes #9234 and #9239 * fix angle conflict and icu dependency * add egl-registry as a dependency * remove space * adding spaces resolved the problem * move files only if they exist * Update the Wrapper to use the Postgres target fixes some observed linkage issues * update baseline * add fontconfig on linux so CI is less flaky in a full rebuild * fix linkage dependent qt5-imageformats libs * Fix Wrapper for Linux and add other platform libs to Qt5::Core * fix plugin properties in cmake files * fix cmake files. * fix missing plugin qminimal deployment into tools dir * change wrapper slightly * UNIX also means APPLE so it needs to be excluded * fix the static windows build * mark the parts of fixcmake which require further work to work with single configuration builds * Update ci.baseline.txt make qt5-tools pass for CI testing * fix last regression * break auto fontconfig in configure script to fix qt5-tools * update baseline * enable verbose to debug ci * try to help it instead of breaking it * completly link icu * try to fix fontconfig configure * fix configure call. cannot be called with a list! * remove invalid line from patch * force icu and reduce the required icu libs according to the configure script * fix icu linkage * add more icu dependencies and names for windows * add more icu libs. Seems like all are needed * fix typo * print icu libs in debug * check releasenames * try again * change link order * add icu to wrapper * fix typo * patch icu configure for static windows builds * add other icu libraries to the icu patch * fix icu in x64-windows-static * update baseline. VTK builds in local WSL so it should work in CI * update VTK control to force rebuild * remove qt5-tools=pass from baseline * remove qcustomplot:x64-linux=fail from baseline Co-authored-by: Robert Schumacher <roschuma@microsoft.com>
89 lines
4.8 KiB
Python
89 lines
4.8 KiB
Python
import os
|
|
import re
|
|
import sys
|
|
from glob import glob
|
|
|
|
port="qt5"
|
|
if len(sys.argv) > 1:
|
|
port=sys.argv[1]
|
|
|
|
files = [y for x in os.walk('.') for y in glob(os.path.join(x[0], '*.cmake'))]
|
|
tooldir="/tools/"+port+"/bin/"
|
|
|
|
for f in files:
|
|
openedfile = open(f, "r")
|
|
builder = ""
|
|
dllpatterndebug = re.compile("_install_prefix}/bin/Qt5.*d+(.dll|.so)")
|
|
libpatterndebug = re.compile("_install_prefix}/lib/Qt5.*d+(.lib|.a)")
|
|
exepattern = re.compile("_install_prefix}/bin/[a-z]+(.exe|)")
|
|
toolexepattern = re.compile("_install_prefix}/tools/qt5/bin/[a-z]+(.exe|)")
|
|
tooldllpattern = re.compile("_install_prefix}/tools/qt5/bin/Qt5.*d+(.dll|.so)")
|
|
populatepluginpattern = re.compile("_populate_[^_]+_plugin_properties\([^ ]+ RELEASE")
|
|
populatetargetpattern = re.compile("_populate_[^_]+_target_properties\(RELEASE ")
|
|
for line in openedfile:
|
|
if "_install_prefix}/tools/qt5/${LIB_LOCATION}" in line:
|
|
builder += " if (${Configuration} STREQUAL \"RELEASE\")"
|
|
builder += "\n " + line.replace("/tools/qt5/bin", "/bin/")
|
|
builder += " else()" #This requires a release and debug build since Qt will check that the file exists!
|
|
#It would be better to use an elseif here with a EXISTS check but that requires a more complicated regex to build the complete filepath since each module uses its own _(qtmodule)_install_prefix
|
|
#so single configuration builds of Qt are currently not supported. Thus =>
|
|
#TODO: Make single configuration builds of Qt work correctly!
|
|
builder += "\n " + line.replace("/tools/qt5/debug/bin", "/debug/bin/")
|
|
builder += " endif()\n"
|
|
elif "_install_prefix}/bin/${LIB_LOCATION}" in line:
|
|
builder += " if (${Configuration} STREQUAL \"RELEASE\")"
|
|
builder += "\n " + line
|
|
builder += " else()" #This requires a release and debug build!
|
|
builder += "\n " + line.replace("/bin/", "/debug/bin/")
|
|
builder += " endif()\n"
|
|
elif "_install_prefix}/lib/${LIB_LOCATION}" in line:
|
|
builder += " if (${Configuration} STREQUAL \"RELEASE\")"
|
|
builder += "\n " + line
|
|
builder += " else()" #This requires a release and debug build!
|
|
builder += "\n " + line.replace("/lib/", "/debug/lib/")
|
|
builder += " endif()\n"
|
|
elif "_install_prefix}/lib/${IMPLIB_LOCATION}" in line:
|
|
builder += " if (${Configuration} STREQUAL \"RELEASE\")"
|
|
builder += "\n " + line
|
|
builder += " else()" #This requires a release and debug build!
|
|
builder += "\n " + line.replace("/lib/", "/debug/lib/")
|
|
builder += " endif()\n"
|
|
elif "_install_prefix}/plugins/${PLUGIN_LOCATION}" in line:
|
|
builder += " if (${Configuration} STREQUAL \"RELEASE\")"
|
|
builder += "\n " + line
|
|
builder += " else()" #This requires a release and debug build!
|
|
builder += "\n " + line.replace("/plugins/", "/debug/plugins/")
|
|
builder += " endif()\n"
|
|
elif "_install_prefix}/lib/qtmaind.lib" in line:
|
|
# qtmaind.lib has been moved to manual-link:
|
|
builder += line.replace("/lib/", "/debug/lib/manual-link/")
|
|
elif "_install_prefix}/lib/qtmain.lib" in line:
|
|
# qtmain(d).lib has been moved to manual-link:
|
|
builder += line.replace("/lib/", "/lib/manual-link/")
|
|
builder += " set(imported_location_debug \"${_qt5Core_install_prefix}/debug/lib/manual-link/qtmaind.lib\")\n"
|
|
builder += "\n"
|
|
builder += " set_target_properties(Qt5::WinMain PROPERTIES\n"
|
|
builder += " IMPORTED_LOCATION_DEBUG ${imported_location_debug}\n"
|
|
builder += " )\n"
|
|
elif populatepluginpattern.search(line) != None:
|
|
builder += line
|
|
builder += line.replace("RELEASE", "DEBUG").replace(".dll", "d.dll").replace(".lib", "d.lib")
|
|
elif populatetargetpattern.search(line) != None:
|
|
builder += line
|
|
builder += line.replace("RELEASE", "DEBUG").replace(".dll", "d.dll").replace(".lib", "d.lib")
|
|
elif dllpatterndebug.search(line) != None:
|
|
builder += line.replace("/bin/", "/debug/bin/")
|
|
elif libpatterndebug.search(line) != None:
|
|
builder += line.replace("/lib/", "/debug/lib/")
|
|
elif tooldllpattern.search(line) != None:
|
|
builder += line.replace("/tools/qt5/bin", "/debug/bin/")
|
|
elif exepattern.search(line) != None:
|
|
builder += line.replace("/bin/", tooldir)
|
|
elif toolexepattern.search(line) != None:
|
|
builder += line.replace("/tools/qt5/bin/",tooldir)
|
|
else:
|
|
builder += line
|
|
new_file = open(f, "w")
|
|
new_file.write(builder)
|
|
new_file.close()
|