mirror of
https://github.com/microsoft/vcpkg.git
synced 2024-11-24 16:39:07 +08:00
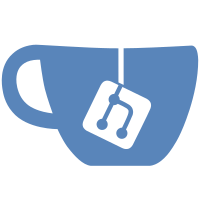
* [ffmpeg] add openh264 support * [ffmpeg] bump port version * [ffmpeg] x-add-version Co-authored-by: Billy Robert ONeal III <bion@microsoft.com>
459 lines
16 KiB
CMake
459 lines
16 KiB
CMake
# Distributed under the OSI-approved BSD 3-Clause License.
|
|
#
|
|
#.rst:
|
|
# FindFFMPEG
|
|
# --------
|
|
#
|
|
# Find the FFPMEG libraries
|
|
#
|
|
# Result Variables
|
|
# ^^^^^^^^^^^^^^^^
|
|
#
|
|
# The following variables will be defined:
|
|
#
|
|
# ``FFMPEG_FOUND``
|
|
# True if FFMPEG found on the local system
|
|
#
|
|
# ``FFMPEG_INCLUDE_DIRS``
|
|
# Location of FFMPEG header files
|
|
#
|
|
# ``FFMPEG_LIBRARY_DIRS``
|
|
# Location of FFMPEG libraries
|
|
#
|
|
# ``FFMPEG_LIBRARIES``
|
|
# List of the FFMPEG libraries found
|
|
#
|
|
#
|
|
|
|
include(FindPackageHandleStandardArgs)
|
|
include(SelectLibraryConfigurations)
|
|
include(CMakeFindDependencyMacro)
|
|
|
|
if(NOT FFMPEG_FOUND)
|
|
|
|
# Compute the installation path relative to this file.
|
|
get_filename_component(SEARCH_PATH "${CMAKE_CURRENT_LIST_FILE}" PATH)
|
|
get_filename_component(SEARCH_PATH "${SEARCH_PATH}" PATH)
|
|
get_filename_component(SEARCH_PATH "${SEARCH_PATH}" PATH)
|
|
if(SEARCH_PATH STREQUAL "/")
|
|
set(SEARCH_PATH "")
|
|
endif()
|
|
|
|
function(select_library_configurations_from_names)
|
|
cmake_parse_arguments(_slc "" "BASENAME" "NAMES;NAMES_RELEASE;NAMES_DEBUG" ${ARGN})
|
|
list(APPEND _slc_NAMES_RELEASE ${_slc_NAMES})
|
|
list(APPEND _slc_NAMES_DEBUG ${_slc_NAMES})
|
|
find_library(${_slc_BASENAME}_LIBRARY_RELEASE NAMES ${_slc_NAMES_RELEASE} PATHS ${SEARCH_PATH}/lib/ NO_DEFAULT_PATH)
|
|
find_library(${_slc_BASENAME}_LIBRARY_DEBUG NAMES ${_slc_NAMES_DEBUG} PATHS ${SEARCH_PATH}/debug/lib/ NO_DEFAULT_PATH)
|
|
select_library_configurations(${_slc_BASENAME})
|
|
set(${_slc_BASENAME}_LIBRARIES ${${_slc_BASENAME}_LIBRARIES} PARENT_SCOPE)
|
|
endfunction()
|
|
|
|
function(select_library_configurations_from_targets)
|
|
cmake_parse_arguments(_slc "" "BASENAME" "TARGETS" ${ARGN})
|
|
foreach(_target ${_slc_TARGETS})
|
|
get_target_property(_rel ${_target} IMPORTED_LOCATION_RELEASE)
|
|
get_target_property(_dbg ${_target} IMPORTED_LOCATION_DEBUG)
|
|
get_target_property(_deps ${_target} INTERFACE_LINK_LIBRARIES)
|
|
list(APPEND ${_slc_BASENAME}_LIBRARY_RELEASE ${_rel})
|
|
list(APPEND ${_slc_BASENAME}_LIBRARY_DEBUG ${_dbg})
|
|
foreach(_dep ${_deps})
|
|
if(TARGET ${_dep})
|
|
get_target_property(_dep_rel ${_dep} IMPORTED_LOCATION_RELEASE)
|
|
get_target_property(_dep_dbg ${_dep} IMPORTED_LOCATION_DEBUG)
|
|
if(_dep_rel MATCHES _dep_rel-NOTFOUND)
|
|
set(_dep_rel ${_dep_dbg})
|
|
elseif(_dep_dbg MATCHES _dep_dbg-NOTFOUND)
|
|
set(_dep_dbg ${_dep_rel})
|
|
endif()
|
|
list(APPEND ${_slc_BASENAME}_LIBRARY_RELEASE ${_dep_rel})
|
|
list(APPEND ${_slc_BASENAME}_LIBRARY_DEBUG ${_dep_dbg})
|
|
elseif(NOT ${_dep} MATCHES _deps-NOTFOUND)
|
|
if(${_dep} MATCHES ::)
|
|
#TODO Handle targets contained in cmake generator expressions
|
|
message(STATUS Unhandled dependency ${_slc_BASENAME}: ${_dep})
|
|
else()
|
|
list(APPEND ${_slc_BASENAME}_DEP_LIBRARIES ${_dep})
|
|
endif()
|
|
endif()
|
|
endforeach()
|
|
endforeach()
|
|
select_library_configurations(${_slc_BASENAME})
|
|
list(APPEND ${_slc_BASENAME}_LIBRARIES ${${_slc_BASENAME}_DEP_LIBRARIES})
|
|
set(${_slc_BASENAME}_LIBRARIES ${${_slc_BASENAME}_LIBRARIES} PARENT_SCOPE)
|
|
endfunction()
|
|
|
|
# for finding system libraries (e.g. Apple framework libraries)
|
|
function(find_platform_dependent_libraries)
|
|
cmake_parse_arguments(_fpd "" "" "NAMES" ${ARGN})
|
|
foreach(_name ${_fpd_NAMES})
|
|
find_library(${_name}_LIBRARY ${_name} REQUIRED)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${${_name}_LIBRARY})
|
|
endforeach()
|
|
set(FFMPEG_PLATFORM_DEPENDENT_LIBS ${FFMPEG_PLATFORM_DEPENDENT_LIBS} PARENT_SCOPE)
|
|
endfunction()
|
|
|
|
# for finding system libraries that may not always be available
|
|
function(find_platform_dependent_optional_libraries)
|
|
cmake_parse_arguments(_fpdo "" "" "NAMES" ${ARGN})
|
|
foreach(_name ${_fpdo_NAMES})
|
|
find_library(${_name}_LIBRARY ${_name})
|
|
if(NOT ${_name}_LIBRARY MATCHES ${_name}_LIBRARY-NOTFOUND)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${${_name}_LIBRARY})
|
|
endif()
|
|
endforeach()
|
|
set(FFMPEG_PLATFORM_DEPENDENT_LIBS ${FFMPEG_PLATFORM_DEPENDENT_LIBS} PARENT_SCOPE)
|
|
endfunction()
|
|
|
|
set(FFMPEG_VERSION "@FFMPEG_VERSION@")
|
|
|
|
find_dependency(Threads)
|
|
if(UNIX)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS -pthread)
|
|
endif()
|
|
|
|
if(@ENABLE_ASS@)
|
|
select_library_configurations_from_names(BASENAME ASS NAMES ass libass)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${ASS_LIBRARIES})
|
|
if(NOT @ENABLE_FREETYPE@)
|
|
find_dependency(Freetype)
|
|
select_library_configurations_from_targets(BASENAME freetype TARGETS Freetype::Freetype)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${freetype_LIBRARIES})
|
|
endif()
|
|
if(NOT @ENABLE_FRIBIDI@)
|
|
select_library_configurations_from_names(BASENAME FRIBIDI NAMES fribidi libfribidi)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${FRIBIDI_LIBRARIES})
|
|
endif()
|
|
select_library_configurations_from_names(BASENAME HARFBUZZ NAMES harfbuzz libharfbuzz)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${HARFBUZZ_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_BZIP2@)
|
|
find_dependency(BZip2)
|
|
select_library_configurations_from_targets(BASENAME BZip2 TARGETS BZip2::BZip2)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${BZip2_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_DAV1D@)
|
|
select_library_configurations_from_names(BASENAME DAV1D NAMES dav1d libdav1d)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${DAV1D_LIBRARIES})
|
|
if(UNIX)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS dl)
|
|
endif()
|
|
endif()
|
|
|
|
if(@ENABLE_ICONV@)
|
|
find_dependency(Iconv)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${Iconv_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_ILBC@)
|
|
select_library_configurations_from_names(BASENAME ILBC NAMES ilbc libilbc)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${ILBC_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_FDKAAC@)
|
|
select_library_configurations_from_names(BASENAME FDK NAMES fdk-aac libfdk-aac)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${FDK_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_FONTCONFIG@)
|
|
find_dependency(Fontconfig)
|
|
select_library_configurations_from_targets(BASENAME fontconfig TARGETS Fontconfig::Fontconfig)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${fontconfig_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_FREETYPE@)
|
|
find_dependency(Freetype)
|
|
select_library_configurations_from_targets(BASENAME freetype TARGETS Freetype::Freetype)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${freetype_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_FRIBIDI@)
|
|
select_library_configurations_from_names(BASENAME FRIBIDI NAMES fribidi libfribidi)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${FRIBIDI_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_LZMA@)
|
|
find_dependency(LibLZMA)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${LibLZMA_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_LAME@)
|
|
find_dependency(mp3lame)
|
|
select_library_configurations_from_targets(BASENAME mp3lame TARGETS mp3lame::mp3lame)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${mp3lame_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_MODPLUG@)
|
|
select_library_configurations_from_names(BASENAME MODPLUG NAMES modplug libmodplug)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${MODPLUG_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_NVCODEC@)
|
|
if(UNIX)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS dl)
|
|
endif()
|
|
endif()
|
|
|
|
if(@ENABLE_OPENCL@)
|
|
find_dependency(OpenCL)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${OpenCL_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_OPENGL@)
|
|
if(POLICY CMP0072)
|
|
cmake_policy (SET CMP0072 OLD)
|
|
endif(POLICY CMP0072)
|
|
find_dependency(OpenGL)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${OPENGL_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_OPENH264@)
|
|
select_library_configurations_from_names(BASENAME openh264 NAMES openh264)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${openh264_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_OPENJPEG@)
|
|
find_dependency(OpenJPEG)
|
|
select_library_configurations_from_targets(BASENAME openjpeg TARGETS openjp2)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${openjpeg_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_OPENSSL@)
|
|
find_dependency(OpenSSL)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${OPENSSL_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_OPUS@)
|
|
find_dependency(Opus)
|
|
select_library_configurations_from_targets(BASENAME Opus TARGETS Opus::opus)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${Opus_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_SDL2@)
|
|
find_dependency(SDL2)
|
|
if(TARGET SDL2::SDL2-static)
|
|
select_library_configurations_from_targets(BASENAME SDL2 TARGETS SDL2::SDL2-static)
|
|
else()
|
|
select_library_configurations_from_targets(BASENAME SDL2 TARGETS SDL2::SDL2)
|
|
endif()
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${SDL2_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_SNAPPY@)
|
|
find_dependency(Snappy)
|
|
select_library_configurations_from_targets(BASENAME Snappy TARGETS Snappy::snappy)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${Snappy_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_SOXR@)
|
|
select_library_configurations_from_names(BASENAME SOXR NAMES soxr)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${SOXR_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_SPEEX@)
|
|
if(WIN32)
|
|
select_library_configurations_from_names(BASENAME SPEEX NAMES_RELEASE libspeex NAMES_DEBUG libspeexd)
|
|
else()
|
|
select_library_configurations_from_names(BASENAME SPEEX NAMES speex libspeex)
|
|
endif()
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${SPEEX_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_SSH@)
|
|
find_dependency(libssh)
|
|
select_library_configurations_from_targets(BASENAME libssh TARGETS ssh)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${libssh_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_THEORA@)
|
|
find_dependency(Ogg) # ensure Ogg::ogg is defined as a target
|
|
find_dependency(unofficial-theora)
|
|
select_library_configurations_from_targets(BASENAME THEORA TARGETS unofficial::theora::theoraenc)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${THEORA_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_TESSERACT@)
|
|
find_dependency(Tesseract)
|
|
select_library_configurations_from_targets(BASENAME tesseract TARGETS libtesseract)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${tesseract_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_VORBIS@)
|
|
find_dependency(Vorbis)
|
|
select_library_configurations_from_targets(BASENAME vorbis TARGETS Vorbis::vorbis Vorbis::vorbisenc)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${vorbis_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_VPX@)
|
|
find_dependency(unofficial-libvpx)
|
|
select_library_configurations_from_targets(BASENAME libvpx TARGETS unofficial::libvpx::libvpx)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${libvpx_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_WAVPACK@)
|
|
find_dependency(wavpack)
|
|
select_library_configurations_from_targets(BASENAME wavpack TARGETS WavPack::wavpack)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${wavpack_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_WEBP@)
|
|
find_dependency(WebP)
|
|
select_library_configurations_from_targets(BASENAME webp TARGETS WebP::webp WebP::webpdemux WebP::libwebpmux WebP::webpdecoder)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${webp_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_X264@)
|
|
select_library_configurations_from_names(BASENAME X264 NAMES x264 libx264)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${X264_LIBRARIES})
|
|
if(UNIX)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS dl)
|
|
endif()
|
|
endif()
|
|
|
|
if(@ENABLE_X265@)
|
|
select_library_configurations_from_names(BASENAME X265 NAMES x265 x265-static)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${X265_LIBRARIES})
|
|
if(UNIX)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS dl)
|
|
find_platform_dependent_optional_libraries(NAMES numa)
|
|
endif()
|
|
endif()
|
|
|
|
if(@ENABLE_XML2@)
|
|
find_dependency(LibXml2)
|
|
select_library_configurations_from_targets(BASENAME libxml2 TARGETS LibXml2::LibXml2)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${libxml2_LIBRARIES})
|
|
endif()
|
|
|
|
if(@ENABLE_ZLIB@)
|
|
find_dependency(ZLIB)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS ${ZLIB_LIBRARIES})
|
|
endif()
|
|
|
|
# Platform dependent libraries required by FFMPEG
|
|
if(UNIX AND NOT APPLE)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS -lX11)
|
|
find_platform_dependent_optional_libraries(NAMES va-drm va vdpau)
|
|
if(@ENABLE_AVDEVICE@)
|
|
find_platform_dependent_optional_libraries(NAMES xcb xcb-shm xcb-shape xcb-xfixes)
|
|
endif()
|
|
endif()
|
|
|
|
if(WIN32)
|
|
if(NOT CYGWIN)
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS wsock32 ws2_32 secur32 bcrypt strmiids Vfw32 Shlwapi mfplat mfuuid)
|
|
endif()
|
|
else()
|
|
list(APPEND FFMPEG_PLATFORM_DEPENDENT_LIBS m)
|
|
endif()
|
|
|
|
macro(FFMPEG_FIND varname shortname headername)
|
|
if(NOT FFMPEG_${varname}_INCLUDE_DIRS)
|
|
find_path(FFMPEG_${varname}_INCLUDE_DIRS NAMES lib${shortname}/${headername} ${headername} PATHS ${SEARCH_PATH}/include NO_DEFAULT_PATH)
|
|
endif()
|
|
if(NOT FFMPEG_${varname}_LIBRARY)
|
|
find_library(FFMPEG_${varname}_LIBRARY_RELEASE NAMES ${shortname} PATHS ${SEARCH_PATH}/lib/ NO_DEFAULT_PATH)
|
|
find_library(FFMPEG_${varname}_LIBRARY_DEBUG NAMES ${shortname}d ${shortname} PATHS ${SEARCH_PATH}/debug/lib/ NO_DEFAULT_PATH)
|
|
get_filename_component(FFMPEG_${varname}_LIBRARY_RELEASE_DIR ${FFMPEG_${varname}_LIBRARY_RELEASE} DIRECTORY)
|
|
get_filename_component(FFMPEG_${varname}_LIBRARY_DEBUG_DIR ${FFMPEG_${varname}_LIBRARY_DEBUG} DIRECTORY)
|
|
select_library_configurations(FFMPEG_${varname})
|
|
set(FFMPEG_${varname}_LIBRARY ${FFMPEG_${varname}_LIBRARY} CACHE STRING "")
|
|
endif()
|
|
if (FFMPEG_${varname}_LIBRARY AND FFMPEG_${varname}_INCLUDE_DIRS)
|
|
set(FFMPEG_${varname}_FOUND TRUE BOOL)
|
|
list(APPEND FFMPEG_INCLUDE_DIRS ${FFMPEG_${varname}_INCLUDE_DIRS})
|
|
list(APPEND FFMPEG_LIBRARIES ${FFMPEG_${varname}_LIBRARY})
|
|
list(APPEND FFMPEG_LIBRARY_DIRS ${FFMPEG_${varname}_LIBRARY_RELEASE_DIR} ${FFMPEG_${varname}_LIBRARY_DEBUG_DIR})
|
|
endif()
|
|
endmacro(FFMPEG_FIND)
|
|
|
|
if(APPLE)
|
|
find_platform_dependent_libraries(NAMES VideoToolbox CoreServices CoreMedia CoreVideo)
|
|
if(@ENABLE_OPENCL@)
|
|
find_platform_dependent_libraries(NAMES OpenCL)
|
|
endif()
|
|
if(@ENABLE_AVDEVICE@)
|
|
find_platform_dependent_libraries(NAMES AVFoundation CoreFoundation CoreGraphics Foundation)
|
|
endif()
|
|
if(@ENABLE_AVFILTER@)
|
|
find_platform_dependent_libraries(NAMES OpenGL AppKit CoreImage)
|
|
endif()
|
|
if(@ENABLE_AVFORMAT@)
|
|
find_platform_dependent_libraries(NAMES Security)
|
|
endif()
|
|
if(@ENABLE_AVCODEC@)
|
|
find_platform_dependent_libraries(NAMES AudioToolbox)
|
|
endif()
|
|
endif()
|
|
|
|
if(@ENABLE_AVDEVICE@)
|
|
FFMPEG_FIND(libavdevice avdevice avdevice.h)
|
|
endif()
|
|
if(@ENABLE_AVFILTER@)
|
|
FFMPEG_FIND(libavfilter avfilter avfilter.h)
|
|
endif()
|
|
if(@ENABLE_AVFORMAT@)
|
|
FFMPEG_FIND(libavformat avformat avformat.h)
|
|
endif()
|
|
if(@ENABLE_AVCODEC@)
|
|
FFMPEG_FIND(libavcodec avcodec avcodec.h)
|
|
endif()
|
|
if(@ENABLE_AVRESAMPLE@)
|
|
FFMPEG_FIND(libavresample avresample avresample.h)
|
|
endif()
|
|
if(@ENABLE_POSTPROC@)
|
|
FFMPEG_FIND(libpostproc postproc postprocess.h)
|
|
endif()
|
|
if(@ENABLE_SWRESAMPLE@)
|
|
FFMPEG_FIND(libswresample swresample swresample.h)
|
|
endif()
|
|
if(@ENABLE_SWSCALE@)
|
|
FFMPEG_FIND(libswscale swscale swscale.h)
|
|
endif()
|
|
FFMPEG_FIND(libavutil avutil avutil.h)
|
|
|
|
if (FFMPEG_libavutil_FOUND)
|
|
list(REMOVE_DUPLICATES FFMPEG_INCLUDE_DIRS)
|
|
list(REMOVE_DUPLICATES FFMPEG_LIBRARY_DIRS)
|
|
set(FFMPEG_libavutil_VERSION "@LIBAVUTIL_VERSION@" CACHE STRING "")
|
|
|
|
if(FFMPEG_libavcodec_FOUND)
|
|
set(FFMPEG_libavcodec_VERSION "@LIBAVCODEC_VERSION@" CACHE STRING "")
|
|
endif()
|
|
if(FFMPEG_libavdevice_FOUND)
|
|
set(FFMPEG_libavdevice_VERSION "@LIBAVDEVICE_VERSION@" CACHE STRING "")
|
|
endif()
|
|
if(FFMPEG_libavfilter_FOUND)
|
|
set(FFMPEG_libavfilter_VERSION "@LIBAVFILTER_VERSION@" CACHE STRING "")
|
|
endif()
|
|
if(FFMPEG_libavformat_FOUND)
|
|
set(FFMPEG_libavformat_VERSION "@LIBAVFORMAT_VERSION@" CACHE STRING "")
|
|
endif()
|
|
if(FFMPEG_libavresample_FOUND)
|
|
set(FFMPEG_libavresample_VERSION "@LIBAVRESAMPLE_VERSION@" CACHE STRING "")
|
|
endif()
|
|
if(FFMPEG_libswresample_FOUND)
|
|
set(FFMPEG_libswresample_VERSION "@LIBSWRESAMPLE_VERSION@" CACHE STRING "")
|
|
endif()
|
|
if(FFMPEG_libswscale_FOUND)
|
|
set(FFMPEG_libswscale_VERSION "@LIBSWSCALE_VERSION@" CACHE STRING "")
|
|
endif()
|
|
|
|
list(APPEND FFMPEG_LIBRARIES
|
|
${FFMPEG_PLATFORM_DEPENDENT_LIBS}
|
|
)
|
|
|
|
set(FFMPEG_LIBRARY ${FFMPEG_LIBRARIES})
|
|
|
|
set(FFMPEG_FOUND TRUE CACHE BOOL "")
|
|
set(FFMPEG_LIBRARIES ${FFMPEG_LIBRARIES} CACHE STRING "")
|
|
set(FFMPEG_INCLUDE_DIRS ${FFMPEG_INCLUDE_DIRS} CACHE STRING "")
|
|
set(FFMPEG_LIBRARY_DIRS ${FFMPEG_LIBRARY_DIRS} CACHE STRING "")
|
|
endif()
|
|
|
|
find_package_handle_standard_args(FFMPEG REQUIRED_VARS FFMPEG_LIBRARIES FFMPEG_LIBRARY_DIRS FFMPEG_INCLUDE_DIRS)
|
|
|
|
endif()
|