mirror of
https://github.com/microsoft/vcpkg.git
synced 2024-11-24 20:32:00 +08:00
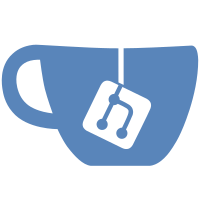
* [vcpkg] Implement constraints in manifests * [vcpkg] Add SourceControlFile::check_against_feature_flags to prevent accidentally ignoring versioning fields * [vcpkg] Switch check_against_feature_flags to accept fs::path * [vcpkg] Implement overrides parsing in manifests * [vcpkg] Address CR comments * [vcpkg] Initial implementation of create_versioned_install_plan() * [vcpkg] Implement port-version minimums * [vcpkg] Implement relaxation phase * [vcpkg] Refactor tests to use check_name_and_version * [vcpkg] Implemented simple relaxed scheme * [vcpkg] More relaxed scheme tests * [vcpkg] Mixed scheme testing * [vcpkg] Support versions and features without defaults * [vcpkg] Support versions and features without defaults 2 * [vcpkg] Only consider greater of toplevel and baseilne * [vcpkg] Implement overrides * [vcpkg] Install defaults * [vcpkg] Handle defaults of transitive packages * [vcpkg] Fix warnings for Span of initializer_list * [vcpkg] Use CMakeVarProvider during versioned install * [vcpkg] Handle inter-feature dependencies * [vcpkg] Correctly handle qualified Dependencies at toplevel * [vcpkg] Address CR comments Co-authored-by: Robert Schumacher <roschuma@microsoft.com>
43 lines
1.6 KiB
C++
43 lines
1.6 KiB
C++
#pragma once
|
|
|
|
#include <vcpkg/cmakevars.h>
|
|
|
|
namespace vcpkg::Test
|
|
{
|
|
struct MockCMakeVarProvider : CMakeVars::CMakeVarProvider
|
|
{
|
|
using SMap = std::unordered_map<std::string, std::string>;
|
|
void load_generic_triplet_vars(Triplet triplet) const override
|
|
{
|
|
generic_triplet_vars.emplace(triplet, SMap{});
|
|
}
|
|
|
|
void load_dep_info_vars(Span<const PackageSpec> specs) const override
|
|
{
|
|
for (auto&& spec : specs)
|
|
dep_info_vars.emplace(spec, SMap{});
|
|
}
|
|
|
|
void load_tag_vars(Span<const FullPackageSpec> specs,
|
|
const PortFileProvider::PortFileProvider& port_provider) const override
|
|
{
|
|
for (auto&& spec : specs)
|
|
tag_vars.emplace(spec.package_spec, SMap{});
|
|
(void)(port_provider);
|
|
}
|
|
|
|
Optional<const std::unordered_map<std::string, std::string>&> get_generic_triplet_vars(
|
|
Triplet triplet) const override;
|
|
|
|
Optional<const std::unordered_map<std::string, std::string>&> get_dep_info_vars(
|
|
const PackageSpec& spec) const override;
|
|
|
|
Optional<const std::unordered_map<std::string, std::string>&> get_tag_vars(
|
|
const PackageSpec& spec) const override;
|
|
|
|
mutable std::unordered_map<PackageSpec, std::unordered_map<std::string, std::string>> dep_info_vars;
|
|
mutable std::unordered_map<PackageSpec, std::unordered_map<std::string, std::string>> tag_vars;
|
|
mutable std::unordered_map<Triplet, std::unordered_map<std::string, std::string>> generic_triplet_vars;
|
|
};
|
|
}
|