mirror of
https://github.com/microsoft/vcpkg.git
synced 2024-12-02 18:51:39 +08:00
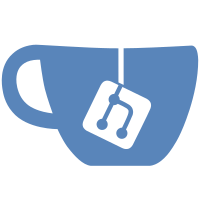
* Add meson from https://github.com/microsoft/vcpkg/pull/12860/ * Add autoconf-archive from https://github.com/microsoft/vcpkg/pull/13081/ * Add kf5windowsystem libs from https://github.com/microsoft/vcpkg/pull/13467/ * Open the FTP and SFTP ports from https://github.com/microsoft/vcpkg/pull/14412/ * Add libxcb-util0-dev from https://github.com/microsoft/vcpkg/pull/14678/ * Add libasound2-dev from https://github.com/microsoft/vcpkg/pull/14774 * Remove no longer necessary apt-mark calls. * Update nasm on Linux. * Fix longstanding bug where Storage was publicly accessible and change to generate SAS token rather than File Share * Delete no longer necessary azure storage firewall rules. * Install the newer Windows SDK with the VS installer instead of manually. * Install the VS2015 and VS2017 compilers. * Update Powershell-Core to 7.1.0. * Update source of WDK. * Update pools. * [opentracing] Repair arm64-windows failures caused by mojibake in `expected.hpp` and errors in opentracing-cpp's lint for arm64 where it thinks exceptions are disabled when they are enabled. Fixes: C:\Dev\vcpkg\buildtrees\opentracing\src\b67575dab0-0250653c81.clean\3rd_party\include\opentracing/expected/expected.hpp(1): warning C4828: The file contains a character starting at offset 0x4a77 that is illegal in the current source character set (codepage 65001). Fixes: D:\buildtrees\opentracing\src\b67575dab0-0250653c81.clean\include\opentracing/tracer.h:223:5: error: cannot use 'try' with exceptions disabled [clang-diagnostic-error] try { ^ * [mmloader] Patch out overrides of CMAKE_C_FLAGS and CMAKE_CXX_FLAGS that inserted /WX. Note that this port sets /GS-, possibly because it may be for authoring shellcode. * Bump storage API version to 2020-04-08.
379 lines
12 KiB
PowerShell
379 lines
12 KiB
PowerShell
# Copyright (c) Microsoft Corporation.
|
|
# SPDX-License-Identifier: MIT
|
|
#
|
|
|
|
<#
|
|
.SYNOPSIS
|
|
Creates a Windows virtual machine scale set, set up for vcpkg's CI.
|
|
|
|
.DESCRIPTION
|
|
create-vmss.ps1 creates an Azure Windows VM scale set, set up for vcpkg's CI
|
|
system. See https://docs.microsoft.com/en-us/azure/virtual-machine-scale-sets/overview
|
|
for more information.
|
|
|
|
This script assumes you have installed Azure tools into PowerShell by following the instructions
|
|
at https://docs.microsoft.com/en-us/powershell/azure/install-az-ps?view=azps-3.6.1
|
|
or are running from Azure Cloud Shell.
|
|
|
|
.PARAMETER Unstable
|
|
If this parameter is set, the machine is configured for use in the "unstable" pool used for testing
|
|
the compiler rather than for testing vcpkg. Differences:
|
|
* The machine prefix is changed to VcpkgUnstable instead of PrWin.
|
|
* No storage account or "archives" share is provisioned.
|
|
* The firewall is not opened to allow communication with Azure Storage.
|
|
#>
|
|
|
|
[CmdLetBinding()]
|
|
Param(
|
|
[switch]$Unstable = $false
|
|
)
|
|
|
|
$Location = 'westus2'
|
|
if ($Unstable) {
|
|
$Prefix = 'VcpkgUnstable-'
|
|
} else {
|
|
$Prefix = 'PrWin-'
|
|
}
|
|
|
|
$Prefix += (Get-Date -Format 'yyyy-MM-dd')
|
|
$VMSize = 'Standard_D16a_v4'
|
|
$ProtoVMName = 'PROTOTYPE'
|
|
$LiveVMPrefix = 'BUILD'
|
|
$WindowsServerSku = '2019-Datacenter'
|
|
$ErrorActionPreference = 'Stop'
|
|
|
|
$ProgressActivity = 'Creating Scale Set'
|
|
$TotalProgress = 12
|
|
if ($Unstable) {
|
|
$TotalProgress -= 1 # skipping the archives share part
|
|
}
|
|
|
|
$CurrentProgress = 1
|
|
|
|
Import-Module "$PSScriptRoot/../create-vmss-helpers.psm1" -DisableNameChecking
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Creating resource group' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
$ResourceGroupName = Find-ResourceGroupName $Prefix
|
|
$AdminPW = New-Password
|
|
New-AzResourceGroup -Name $ResourceGroupName -Location $Location
|
|
$AdminPWSecure = ConvertTo-SecureString $AdminPW -AsPlainText -Force
|
|
$Credential = New-Object System.Management.Automation.PSCredential ("AdminUser", $AdminPWSecure)
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Creating virtual network' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
$allFirewallRules = @()
|
|
|
|
$allFirewallRules += New-AzNetworkSecurityRuleConfig `
|
|
-Name AllowHTTP `
|
|
-Description 'Allow HTTP(S)' `
|
|
-Access Allow `
|
|
-Protocol Tcp `
|
|
-Direction Outbound `
|
|
-Priority 1008 `
|
|
-SourceAddressPrefix * `
|
|
-SourcePortRange * `
|
|
-DestinationAddressPrefix * `
|
|
-DestinationPortRange @(80, 443)
|
|
|
|
$allFirewallRules += New-AzNetworkSecurityRuleConfig `
|
|
-Name AllowSFTP `
|
|
-Description 'Allow (S)FTP' `
|
|
-Access Allow `
|
|
-Protocol Tcp `
|
|
-Direction Outbound `
|
|
-Priority 1009 `
|
|
-SourceAddressPrefix * `
|
|
-SourcePortRange * `
|
|
-DestinationAddressPrefix * `
|
|
-DestinationPortRange @(21, 22)
|
|
|
|
$allFirewallRules += New-AzNetworkSecurityRuleConfig `
|
|
-Name AllowDNS `
|
|
-Description 'Allow DNS' `
|
|
-Access Allow `
|
|
-Protocol * `
|
|
-Direction Outbound `
|
|
-Priority 1010 `
|
|
-SourceAddressPrefix * `
|
|
-SourcePortRange * `
|
|
-DestinationAddressPrefix * `
|
|
-DestinationPortRange 53
|
|
|
|
$allFirewallRules += New-AzNetworkSecurityRuleConfig `
|
|
-Name AllowGit `
|
|
-Description 'Allow git' `
|
|
-Access Allow `
|
|
-Protocol Tcp `
|
|
-Direction Outbound `
|
|
-Priority 1011 `
|
|
-SourceAddressPrefix * `
|
|
-SourcePortRange * `
|
|
-DestinationAddressPrefix * `
|
|
-DestinationPortRange 9418
|
|
|
|
$allFirewallRules += New-AzNetworkSecurityRuleConfig `
|
|
-Name DenyElse `
|
|
-Description 'Deny everything else' `
|
|
-Access Deny `
|
|
-Protocol * `
|
|
-Direction Outbound `
|
|
-Priority 1013 `
|
|
-SourceAddressPrefix * `
|
|
-SourcePortRange * `
|
|
-DestinationAddressPrefix * `
|
|
-DestinationPortRange *
|
|
|
|
$NetworkSecurityGroupName = $ResourceGroupName + 'NetworkSecurity'
|
|
$NetworkSecurityGroup = New-AzNetworkSecurityGroup `
|
|
-Name $NetworkSecurityGroupName `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Location $Location `
|
|
-SecurityRules $allFirewallRules
|
|
|
|
$SubnetName = $ResourceGroupName + 'Subnet'
|
|
$Subnet = New-AzVirtualNetworkSubnetConfig `
|
|
-Name $SubnetName `
|
|
-AddressPrefix "10.0.0.0/16" `
|
|
-NetworkSecurityGroup $NetworkSecurityGroup `
|
|
-ServiceEndpoint "Microsoft.Storage"
|
|
|
|
$VirtualNetworkName = $ResourceGroupName + 'Network'
|
|
$VirtualNetwork = New-AzVirtualNetwork `
|
|
-Name $VirtualNetworkName `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Location $Location `
|
|
-AddressPrefix "10.0.0.0/16" `
|
|
-Subnet $Subnet
|
|
|
|
####################################################################################################
|
|
if (-Not $Unstable) {
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Creating archives storage account' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
$StorageAccountName = Sanitize-Name $ResourceGroupName
|
|
|
|
New-AzStorageAccount `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Location $Location `
|
|
-Name $StorageAccountName `
|
|
-SkuName 'Standard_LRS' `
|
|
-Kind StorageV2
|
|
|
|
$StorageAccountKeys = Get-AzStorageAccountKey `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Name $StorageAccountName
|
|
|
|
$StorageAccountKey = $StorageAccountKeys[0].Value
|
|
|
|
$StorageContext = New-AzStorageContext `
|
|
-StorageAccountName $StorageAccountName `
|
|
-StorageAccountKey $StorageAccountKey
|
|
|
|
New-AzStorageContainer -Name archives -Context $StorageContext -Permission Off
|
|
$StartTime = [DateTime]::Now
|
|
$ExpiryTime = $StartTime.AddMonths(6)
|
|
|
|
$SasToken = New-AzStorageAccountSASToken `
|
|
-Service Blob `
|
|
-Permission "racwdlup" `
|
|
-Context $StorageContext `
|
|
-StartTime $StartTime `
|
|
-ExpiryTime $ExpiryTime `
|
|
-ResourceType Service,Container,Object `
|
|
-Protocol HttpsOnly
|
|
|
|
$SasToken = $SasToken.Substring(1) # strip leading ?
|
|
|
|
# Note that we put the storage account into the firewall after creating the above SAS token or we
|
|
# would be denied since the person running this script isn't one of the VMs we're creating here.
|
|
Set-AzStorageAccount `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-AccountName $StorageAccountName `
|
|
-NetworkRuleSet ( `
|
|
@{bypass="AzureServices"; `
|
|
virtualNetworkRules=( `
|
|
@{VirtualNetworkResourceId=$VirtualNetwork.Subnets[0].Id;Action="allow"}); `
|
|
defaultAction="Deny"})
|
|
}
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Creating prototype VM' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
$NicName = $ResourceGroupName + 'NIC'
|
|
$Nic = New-AzNetworkInterface `
|
|
-Name $NicName `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Location $Location `
|
|
-Subnet $VirtualNetwork.Subnets[0]
|
|
|
|
$VM = New-AzVMConfig -Name $ProtoVMName -VMSize $VMSize -Priority 'Spot' -MaxPrice -1
|
|
$VM = Set-AzVMOperatingSystem `
|
|
-VM $VM `
|
|
-Windows `
|
|
-ComputerName $ProtoVMName `
|
|
-Credential $Credential `
|
|
-ProvisionVMAgent
|
|
|
|
$VM = Add-AzVMNetworkInterface -VM $VM -Id $Nic.Id
|
|
$VM = Set-AzVMSourceImage `
|
|
-VM $VM `
|
|
-PublisherName 'MicrosoftWindowsServer' `
|
|
-Offer 'WindowsServer' `
|
|
-Skus $WindowsServerSku `
|
|
-Version latest
|
|
|
|
$VM = Set-AzVMBootDiagnostic -VM $VM -Disable
|
|
New-AzVm `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Location $Location `
|
|
-VM $VM
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Running provisioning script provision-image.txt (as a .ps1) in VM' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
$provisionParameters = @{AdminUserPassword = $AdminPW;}
|
|
if (-Not $Unstable) {
|
|
$provisionParameters['StorageAccountName'] = $StorageAccountName
|
|
$provisionParameters['StorageAccountSasToken'] = $SasToken
|
|
}
|
|
|
|
$ProvisionImageResult = Invoke-AzVMRunCommand `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-VMName $ProtoVMName `
|
|
-CommandId 'RunPowerShellScript' `
|
|
-ScriptPath "$PSScriptRoot\provision-image.txt" `
|
|
-Parameter $provisionParameters
|
|
|
|
Write-Host "provision-image.ps1 output: $($ProvisionImageResult.value.Message)"
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Restarting VM' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
Restart-AzVM -ResourceGroupName $ResourceGroupName -Name $ProtoVMName
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Running provisioning script sysprep.ps1 in VM' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
$SysprepResult = Invoke-AzVMRunCommand `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-VMName $ProtoVMName `
|
|
-CommandId 'RunPowerShellScript' `
|
|
-ScriptPath "$PSScriptRoot\sysprep.ps1"
|
|
|
|
Write-Host "sysprep.ps1 output: $($SysprepResult.value.Message)"
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Waiting for VM to shut down' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
Wait-Shutdown -ResourceGroupName $ResourceGroupName -Name $ProtoVMName
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Converting VM to Image' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
Stop-AzVM `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Name $ProtoVMName `
|
|
-Force
|
|
|
|
Set-AzVM `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Name $ProtoVMName `
|
|
-Generalized
|
|
|
|
$VM = Get-AzVM -ResourceGroupName $ResourceGroupName -Name $ProtoVMName
|
|
$PrototypeOSDiskName = $VM.StorageProfile.OsDisk.Name
|
|
$ImageConfig = New-AzImageConfig -Location $Location -SourceVirtualMachineId $VM.ID
|
|
$Image = New-AzImage -Image $ImageConfig -ImageName $ProtoVMName -ResourceGroupName $ResourceGroupName
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Deleting unused VM and disk' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
Remove-AzVM -Id $VM.ID -Force
|
|
Remove-AzDisk -ResourceGroupName $ResourceGroupName -DiskName $PrototypeOSDiskName -Force
|
|
|
|
####################################################################################################
|
|
Write-Progress `
|
|
-Activity $ProgressActivity `
|
|
-Status 'Creating scale set' `
|
|
-PercentComplete (100 / $TotalProgress * $CurrentProgress++)
|
|
|
|
$VmssIpConfigName = $ResourceGroupName + 'VmssIpConfig'
|
|
$VmssIpConfig = New-AzVmssIpConfig -SubnetId $Nic.IpConfigurations[0].Subnet.Id -Primary -Name $VmssIpConfigName
|
|
$VmssName = $ResourceGroupName + 'Vmss'
|
|
$Vmss = New-AzVmssConfig `
|
|
-Location $Location `
|
|
-SkuCapacity 0 `
|
|
-SkuName $VMSize `
|
|
-SkuTier 'Standard' `
|
|
-Overprovision $false `
|
|
-UpgradePolicyMode Manual `
|
|
-EvictionPolicy Delete `
|
|
-Priority Spot `
|
|
-MaxPrice -1
|
|
|
|
$Vmss = Add-AzVmssNetworkInterfaceConfiguration `
|
|
-VirtualMachineScaleSet $Vmss `
|
|
-Primary $true `
|
|
-IpConfiguration $VmssIpConfig `
|
|
-NetworkSecurityGroupId $NetworkSecurityGroup.Id `
|
|
-Name $NicName
|
|
|
|
$Vmss = Set-AzVmssOsProfile `
|
|
-VirtualMachineScaleSet $Vmss `
|
|
-ComputerNamePrefix $LiveVMPrefix `
|
|
-AdminUsername 'AdminUser' `
|
|
-AdminPassword $AdminPW `
|
|
-WindowsConfigurationProvisionVMAgent $true `
|
|
-WindowsConfigurationEnableAutomaticUpdate $true
|
|
|
|
$Vmss = Set-AzVmssStorageProfile `
|
|
-VirtualMachineScaleSet $Vmss `
|
|
-OsDiskCreateOption 'FromImage' `
|
|
-OsDiskCaching ReadWrite `
|
|
-ImageReferenceId $Image.Id
|
|
|
|
New-AzVmss `
|
|
-ResourceGroupName $ResourceGroupName `
|
|
-Name $VmssName `
|
|
-VirtualMachineScaleSet $Vmss
|
|
|
|
####################################################################################################
|
|
Write-Progress -Activity $ProgressActivity -Completed
|
|
Write-Host "Location: $Location"
|
|
Write-Host "Resource group name: $ResourceGroupName"
|
|
Write-Host "User name: AdminUser"
|
|
Write-Host "Using generated password: $AdminPW"
|
|
Write-Host 'Finished!'
|