mirror of
https://github.com/ant-design/ant-design.git
synced 2024-12-05 01:19:45 +08:00
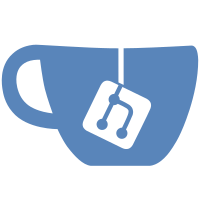
* refactor: Skeleton.Button square shape style the Button's square shape style is same as Avator's that its width is equal to height, and the old square shape become the default shape that its width is double size to height. * fix: `ButtonShapeType` type annotation
3.2 KiB
3.2 KiB
order | title | ||||
---|---|---|---|---|---|
2.1 |
|
zh-CN
骨架按钮、头像、输入框和图像。
en-US
Skeleton Button, Avatar, Input and Image.
import type { RadioChangeEvent } from 'antd';
import { Divider, Form, Radio, Skeleton, Space, Switch } from 'antd';
import React, { useState } from 'react';
type SizeType = 'default' | 'small' | 'large';
type ButtonShapeType = 'circle' | 'square' | 'round' | 'default';
type AvatarShapeType = 'circle' | 'square';
const App: React.FC = () => {
const [active, setActive] = useState(false);
const [block, setBlock] = useState(false);
const [size, setSize] = useState<SizeType>('default');
const [buttonShape, setButtonShape] = useState<ButtonShapeType>('default');
const [avatarShape, setAvatarShape] = useState<AvatarShapeType>('circle');
const handleActiveChange = (checked: boolean) => {
setActive(checked);
};
const handleBlockChange = (checked: boolean) => {
setBlock(checked);
};
const handleSizeChange = (e: RadioChangeEvent) => {
setSize(e.target.value);
};
const handleShapeButton = (e: RadioChangeEvent) => {
setButtonShape(e.target.value);
};
const handleAvatarShape = (e: RadioChangeEvent) => {
setAvatarShape(e.target.value);
};
return (
<>
<Space>
<Skeleton.Button active={active} size={size} shape={buttonShape} block={block} />
<Skeleton.Avatar active={active} size={size} shape={avatarShape} />
<Skeleton.Input active={active} size={size} />
</Space>
<br />
<br />
<Skeleton.Button active={active} size={size} shape={buttonShape} block={block} />
<br />
<br />
<Skeleton.Input active={active} size={size} block={block} />
<br />
<br />
<Skeleton.Image />
<Divider />
<Form layout="inline" style={{ margin: '16px 0' }}>
<Form.Item label="Active">
<Switch checked={active} onChange={handleActiveChange} />
</Form.Item>
<Form.Item label="Button and Input Block">
<Switch checked={block} onChange={handleBlockChange} />
</Form.Item>
<Form.Item label="Size">
<Radio.Group value={size} onChange={handleSizeChange}>
<Radio.Button value="default">Default</Radio.Button>
<Radio.Button value="large">Large</Radio.Button>
<Radio.Button value="small">Small</Radio.Button>
</Radio.Group>
</Form.Item>
<Form.Item label="Button Shape">
<Radio.Group value={buttonShape} onChange={handleShapeButton}>
<Radio.Button value="default">Default</Radio.Button>
<Radio.Button value="square">Square</Radio.Button>
<Radio.Button value="round">Round</Radio.Button>
<Radio.Button value="circle">Circle</Radio.Button>
</Radio.Group>
</Form.Item>
<Form.Item label="Avatar Shape">
<Radio.Group value={avatarShape} onChange={handleAvatarShape}>
<Radio.Button value="square">Square</Radio.Button>
<Radio.Button value="circle">Circle</Radio.Button>
</Radio.Group>
</Form.Item>
</Form>
</>
);
};
export default App;