mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-26 04:00:13 +08:00
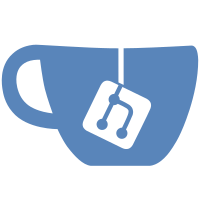
* feat: support Form[layout], close: #5056 * feat: Form should be responsive, close: #5055 * test: update snapshot * fix: resolve conflict betwen layout defaultValue and inline & vertical
107 lines
2.7 KiB
Markdown
107 lines
2.7 KiB
Markdown
---
|
|
order: 4
|
|
title:
|
|
zh-CN: 弹出层中的新建表单
|
|
en-US: Form in Modal to Create
|
|
---
|
|
|
|
## zh-CN
|
|
|
|
当用户访问一个展示了某个列表的页面,想新建一项但又不想跳转页面时,可以用 Modal 弹出一个表单,用户填写必要信息后创建新的项。
|
|
|
|
## en-US
|
|
|
|
When user visit a page with a list of items, and want to create a new item. The page can popup a form in Modal, then let user fills in the form to create an item.
|
|
|
|
````jsx
|
|
import { Button, Modal, Form, Input, Radio } from 'antd';
|
|
const FormItem = Form.Item;
|
|
|
|
const CollectionCreateForm = Form.create()(
|
|
(props) => {
|
|
const { visible, onCancel, onCreate, form } = props;
|
|
const { getFieldDecorator } = form;
|
|
return (
|
|
<Modal
|
|
visible={visible}
|
|
title="Create a new collection"
|
|
okText="Create"
|
|
onCancel={onCancel}
|
|
onOk={onCreate}
|
|
>
|
|
<Form layout="vertical">
|
|
<FormItem label="Title">
|
|
{getFieldDecorator('title', {
|
|
rules: [{ required: true, message: 'Please input the title of collection!' }],
|
|
})(
|
|
<Input />
|
|
)}
|
|
</FormItem>
|
|
<FormItem label="Description">
|
|
{getFieldDecorator('description')(<Input type="textarea" />)}
|
|
</FormItem>
|
|
<FormItem className="collection-create-form_last-form-item">
|
|
{getFieldDecorator('modifier', {
|
|
initialValue: 'public',
|
|
})(
|
|
<Radio.Group>
|
|
<Radio value="public">Public</Radio>
|
|
<Radio value="private">Private</Radio>
|
|
</Radio.Group>
|
|
)}
|
|
</FormItem>
|
|
</Form>
|
|
</Modal>
|
|
);
|
|
}
|
|
);
|
|
|
|
class CollectionsPage extends React.Component {
|
|
state = {
|
|
visible: false,
|
|
};
|
|
showModal = () => {
|
|
this.setState({ visible: true });
|
|
}
|
|
handleCancel = () => {
|
|
this.setState({ visible: false });
|
|
}
|
|
handleCreate = () => {
|
|
const form = this.form;
|
|
form.validateFields((err, values) => {
|
|
if (err) {
|
|
return;
|
|
}
|
|
|
|
console.log('Received values of form: ', values);
|
|
form.resetFields();
|
|
this.setState({ visible: false });
|
|
});
|
|
}
|
|
saveFormRef = (form) => {
|
|
this.form = form;
|
|
}
|
|
render() {
|
|
return (
|
|
<div>
|
|
<Button type="primary" onClick={this.showModal}>New Collection</Button>
|
|
<CollectionCreateForm
|
|
ref={this.saveFormRef}
|
|
visible={this.state.visible}
|
|
onCancel={this.handleCancel}
|
|
onCreate={this.handleCreate}
|
|
/>
|
|
</div>
|
|
);
|
|
}
|
|
}
|
|
|
|
ReactDOM.render(<CollectionsPage />, mountNode);
|
|
````
|
|
|
|
````css
|
|
.collection-create-form_last-form-item {
|
|
margin-bottom: 0;
|
|
}
|
|
````
|