mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-26 04:00:13 +08:00
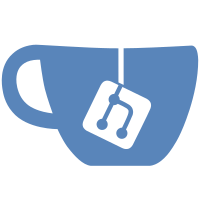
This PR adds the `okButtonProps` and `cancelButtonProps` API to the `Modal.confirm` and associated methods. * [x] Make sure that you propose PR to right branch: bugfix for `master`, feature for branch `feature`. * [x] Make sure that you follow antd's [code convention](https://github.com/ant-design/ant-design/wiki/Code-convention-for-antd). * [x] Run `npm run lint` and fix those errors before submitting in order to keep consistent code style. * [x] Rebase before creating a PR to keep commit history clear. * [x] Add some descriptions and refer relative issues for you PR. Extra checklist: *isNewFeature* **:** * [x] Update API docs for the component. * [x] Update/Add demo to demonstrate new feature. * [x] Update TypeScript definition for the component. * [x] Add unit tests for the feature.
1.5 KiB
1.5 KiB
order | title | ||||
---|---|---|---|---|---|
3 |
|
zh-CN
使用 confirm()
可以快捷地弹出确认框。
en-US
To use confirm()
to popup a confirmation modal dialog.
import { Modal, Button } from 'antd';
const confirm = Modal.confirm;
function showConfirm() {
confirm({
title: 'Do you Want to delete these items?',
content: 'Some descriptions',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}
function showDeleteConfirm() {
confirm({
title: 'Are you sure delete this task?',
content: 'Some descriptions',
okText: 'Yes',
okType: 'danger',
cancelText: 'No',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}
function showPropsConfirm() {
confirm({
title: 'Are you sure delete this task?',
content: 'Some descriptions',
okText: 'Yes',
okType: 'danger',
okButtonProps: {
disabled: true,
},
cancelButtonProps: {
loading: true,
},
cancelText: 'No',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}
ReactDOM.render(
<div>
<Button onClick={showConfirm}>
Confirm
</Button>
<Button onClick={showDeleteConfirm} type="dashed">
Delete
</Button>
<Button onClick={showPropsConfirm} type="dashed">
With extra props
</Button>
</div>,
mountNode);