mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-26 04:00:13 +08:00
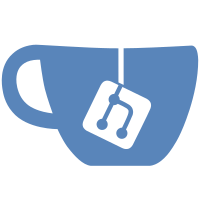
In the example with the editor, the new comments are added on top of the older ones which is not following the way it is usually done in discussions.
2.3 KiB
2.3 KiB
order | title | ||||
---|---|---|---|---|---|
3 |
|
zh-CN
评论编辑器组件提供了相同样式的封装以支持自定义评论编辑器。
en-US
Comment can be used as an editor, so the user can customize the contents of the component.
import { Comment, Avatar, Form, Button, List, Input } from 'antd';
import moment from 'moment';
const { TextArea } = Input;
const CommentList = ({ comments }) => (
<List
dataSource={comments}
header={`${comments.length} ${comments.length > 1 ? 'replies' : 'reply'}`}
itemLayout="horizontal"
renderItem={props => <Comment {...props} />}
/>
);
const Editor = ({ onChange, onSubmit, submitting, value }) => (
<>
<Form.Item>
<TextArea rows={4} onChange={onChange} value={value} />
</Form.Item>
<Form.Item>
<Button htmlType="submit" loading={submitting} onClick={onSubmit} type="primary">
Add Comment
</Button>
</Form.Item>
</>
);
class App extends React.Component {
state = {
comments: [],
submitting: false,
value: '',
};
handleSubmit = () => {
if (!this.state.value) {
return;
}
this.setState({
submitting: true,
});
setTimeout(() => {
this.setState({
submitting: false,
value: '',
comments: [
...this.state.comments,
{
author: 'Han Solo',
avatar: 'https://zos.alipayobjects.com/rmsportal/ODTLcjxAfvqbxHnVXCYX.png',
content: <p>{this.state.value}</p>,
datetime: moment().fromNow(),
},
],
});
}, 1000);
};
handleChange = e => {
this.setState({
value: e.target.value,
});
};
render() {
const { comments, submitting, value } = this.state;
return (
<>
{comments.length > 0 && <CommentList comments={comments} />}
<Comment
avatar={
<Avatar
src="https://zos.alipayobjects.com/rmsportal/ODTLcjxAfvqbxHnVXCYX.png"
alt="Han Solo"
/>
}
content={
<Editor
onChange={this.handleChange}
onSubmit={this.handleSubmit}
submitting={submitting}
value={value}
/>
}
/>
</>
);
}
}
ReactDOM.render(<App />, mountNode);