mirror of
https://github.com/nginx/nginx.git
synced 2024-12-17 23:27:49 +08:00
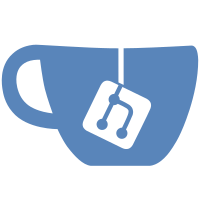
*) Bugfix: the segmentation fault occurred when the signal queue overflowed if the "rtsig" method was used; the bug had appeared in 0.2.0. *) Change: correct handling of the "\\", "\"", "\'", and "\$" pairs in SSI.
86 lines
1.8 KiB
C
86 lines
1.8 KiB
C
|
|
/*
|
|
* Copyright (C) Igor Sysoev
|
|
*/
|
|
|
|
|
|
#ifndef _NGX_PROCESS_H_INCLUDED_
|
|
#define _NGX_PROCESS_H_INCLUDED_
|
|
|
|
|
|
#include <ngx_setproctitle.h>
|
|
|
|
|
|
typedef pid_t ngx_pid_t;
|
|
|
|
#define NGX_INVALID_PID -1
|
|
|
|
typedef void (*ngx_spawn_proc_pt) (ngx_cycle_t *cycle, void *data);
|
|
|
|
typedef struct {
|
|
ngx_pid_t pid;
|
|
int status;
|
|
ngx_socket_t channel[2];
|
|
|
|
ngx_spawn_proc_pt proc;
|
|
void *data;
|
|
char *name;
|
|
|
|
unsigned respawn:1;
|
|
unsigned just_respawn:1;
|
|
unsigned detached:1;
|
|
unsigned exiting:1;
|
|
unsigned exited:1;
|
|
} ngx_process_t;
|
|
|
|
|
|
typedef struct {
|
|
char *path;
|
|
char *name;
|
|
char *const *argv;
|
|
char *const *envp;
|
|
} ngx_exec_ctx_t;
|
|
|
|
|
|
#define NGX_MAX_PROCESSES 1024
|
|
|
|
#define NGX_PROCESS_NORESPAWN -1
|
|
#define NGX_PROCESS_RESPAWN -2
|
|
#define NGX_PROCESS_JUST_RESPAWN -3
|
|
#define NGX_PROCESS_DETACHED -4
|
|
|
|
|
|
#define ngx_getpid getpid
|
|
|
|
#ifndef ngx_log_pid
|
|
#define ngx_log_pid ngx_pid
|
|
#endif
|
|
|
|
|
|
ngx_pid_t ngx_spawn_process(ngx_cycle_t *cycle,
|
|
ngx_spawn_proc_pt proc, void *data, char *name, ngx_int_t respawn);
|
|
ngx_pid_t ngx_execute(ngx_cycle_t *cycle, ngx_exec_ctx_t *ctx);
|
|
ngx_int_t ngx_init_signals(ngx_log_t *log);
|
|
void ngx_debug_point(void);
|
|
|
|
|
|
#if (NGX_HAVE_SCHED_YIELD)
|
|
#define ngx_sched_yield() sched_yield()
|
|
#else
|
|
#define ngx_sched_yield() usleep(1)
|
|
#endif
|
|
|
|
|
|
extern int ngx_argc;
|
|
extern char **ngx_argv;
|
|
extern char **ngx_os_argv;
|
|
|
|
extern ngx_pid_t ngx_pid;
|
|
extern ngx_socket_t ngx_channel;
|
|
extern ngx_int_t ngx_process_slot;
|
|
extern ngx_int_t ngx_last_process;
|
|
extern ngx_process_t ngx_processes[NGX_MAX_PROCESSES];
|
|
|
|
|
|
#endif /* _NGX_PROCESS_H_INCLUDED_ */
|