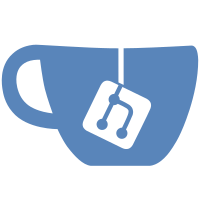
* chore:(core): migrate to tsup * chore: migrate blockquote and bold to tsup * chore: migrated bubble-menu and bullet-list to tsup * chore: migrated more packages to tsup * chore: migrate code and character extensions to tsup * chore: update package.json to simplify build for all packages * chore: move all packages to tsup as a build process * chore: change ci build task * feat(pm): add prosemirror meta package * rfix: resolve issues with build paths & export mappings * docs: update documentation to include notes for @tiptap/pm * chore(pm): update tsconfig * chore(packages): update packages * fix(pm): add package export infos & fix dependencies * chore(general): start moving to pm package as deps * chore: move to tiptap pm package internally * fix(demos): fix demos working with new pm package * fix(tables): fix tables package * fix(tables): fix tables package * chore(demos): pinned typescript version * chore: remove unnecessary tsconfig * chore: fix netlify build * fix(demos): fix package resolving for pm packages * fix(tests): fix package resolving for pm packages * fix(tests): fix package resolving for pm packages * chore(tests): fix tests not running correctly after pm package * chore(pm): add files to files array * chore: update build workflow * chore(tests): increase timeout time back to 12s * chore(docs): update docs * chore(docs): update installation guides & pm information to docs * chore(docs): add link to prosemirror docs * fix(vue-3): add missing build step * chore(docs): comment out cdn link * chore(docs): remove semicolons from docs * chore(docs): remove unnecessary installation note * chore(docs): remove unnecessary installation note
4.3 KiB
title | tableOfContents |
---|---|
Nuxt.js WYSIWYG | true |
Nuxt.js
Introduction
The following guide describes how to integrate Tiptap with your Nuxt.js project.
Requirements
1. Create a project (optional)
If you already have an existing Vue project, that’s fine too. Just skip this step and proceed with the next step.
For the sake of this guide, let’s start with a fresh Nuxt.js project called my-tiptap-project
. The following command sets up everything we need. It asks a lot of questions, but just use what floats your boat or use the defaults.
# create a project
npm init nuxt-app my-tiptap-project
# change directory
cd my-tiptap-project
2. Install the dependencies
Okay, enough of the boring boilerplate work. Let’s finally install Tiptap! For the following example you’ll need the @tiptap/vue-2
package with a few components, the @tiptap/pm
package, and @tiptap/starter-kit
which has the most common extensions to get started quickly.
npm install @tiptap/vue-2 @tiptap/pm @tiptap/starter-kit
If you followed step 1 and 2, you can now start your project with npm run serve
, and open http://localhost:8080/ in your favorite browser. This might be different, if you’re working with an existing project.
3. Create a new component
To actually start using Tiptap, you’ll need to add a new component to your app. Let’s call it TiptapEditor
and put the following example code in components/TiptapEditor.vue
.
This is the fastest way to get Tiptap up and running with Vue. It will give you a very basic version of Tiptap, without any buttons. No worries, you will be able to add more functionality soon.
<template>
<editor-content :editor="editor" />
</template>
<script>
import { Editor, EditorContent } from '@tiptap/vue-2'
import StarterKit from '@tiptap/starter-kit'
export default {
components: {
EditorContent,
},
data() {
return {
editor: null,
}
},
mounted() {
this.editor = new Editor({
content: '<p>I’m running Tiptap with Vue.js. 🎉</p>',
extensions: [
StarterKit,
],
})
},
beforeDestroy() {
this.editor.destroy()
},
}
</script>
4. Add it to your app
Now, let’s replace the content of pages/index.vue
with the following example code to use our new TiptapEditor
component in our app.
<template>
<div>
<client-only>
<tiptap-editor />
</client-only>
</div>
</template>
<script>
import TiptapEditor from '~/components/TiptapEditor.vue'
export default {
components: {
TiptapEditor
}
}
</script>
Note that Tiptap needs to run in the client, not on the server. It’s required to wrap the editor in a <client-only>
tag. Read more about client-only components.
You should now see Tiptap in your browser. Time to give yourself a pat on the back! :)
5. Use v-model (optional)
You’re probably used to bind your data with v-model
in forms, that’s also possible with Tiptap. Here is a working example component, that you can integrate in your project:
https://embed.tiptap.dev/preview/GuideGettingStarted/VModel
<template>
<editor-content :editor="editor" />
</template>
<script>
import { Editor, EditorContent } from '@tiptap/vue-2'
import StarterKit from '@tiptap/starter-kit'
export default {
components: {
EditorContent,
},
props: {
value: {
type: String,
default: '',
},
},
data() {
return {
editor: null,
}
},
watch: {
value(value) {
// HTML
const isSame = this.editor.getHTML() === value
// JSON
// const isSame = JSON.stringify(this.editor.getJSON()) === JSON.stringify(value)
if (isSame) {
return
}
this.editor.commands.setContent(value, false)
},
},
mounted() {
this.editor = new Editor({
content: this.value,
extensions: [
StarterKit,
],
onUpdate: () => {
// HTML
this.$emit('input', this.editor.getHTML())
// JSON
// this.$emit('input', this.editor.getJSON())
},
})
},
beforeDestroy() {
this.editor.destroy()
},
}
</script>